Hello !
In this tutorial , you’ll find out how to create your own web service using the Slim Framework and why it’s important to create a web service.
Let’s get started !
if you are familiar with the web services terms , you can skip the definitions and start developing your first web service here .
What’s a web service ?
A web service is a secure way to offer the database data to other applications .I’d like to illustrate my point with an example : Imagine you have a database that contains many personal data of your users and you would like to give access to some data to other developers, How can you do it ?
Without the web services , you have to give the developers the database server address , the database login and password , then the developers will have full access to your database which is a bad solution !
💡 The solution is creating a web service that offers only the data needed , so the developers will not get your database credentials thus they will not have full access to your database .
You get the point .
Introduction to Slim Framework
Slim framework is a great PHP framework that will let you create your own web service without spending too much time .It’s open source and well documented .
JSON
To put it simply , JSON is a text based format used to transmit data between two different apps . So , our data will be structured in a universal format in such a way that others apps will be able to process it easily .
Too much annoying details ? So let’s start developing our first web service .
Develop your web service 😎
-
Getting the data (JSON)
First, we should define our route , in this example when we visit yourdomain/cars it will call the method ‘getCars’
$app->get('/cars','getCars');
Then we should define our ‘getCars’ method which will return all the cars contained in the Database .To do that , we have to write our Sql query
$sql = "SELECT manufacturer,color FROM car ORDER BY id";
Then , execute the query
$db = getDB();
$stmt = $db->query($sql);
$cars = $stmt->fetchAll(PDO::FETCH_OBJ);
and finally return the data as JSON with this line of code
echo '{"Cars": ' . json_encode($cars) . '}';
The ‘getCars’ method will be :
function getCars() {
$sql = "SELECT manufacturer,color FROM car ORDER BY id";
try {
$db = getDB();
$stmt = $db->query($sql);
$cars = $stmt->fetchAll(PDO::FETCH_OBJ);
$db = null;
echo '{"Cars": ' . json_encode($cars) . '}';
} catch(PDOException $e) {
echo "Error !";
}
}
Let’s test it ! go to the browser and visit yourdomain/cars , it will show you this
{"Cars": [{"manufacturer":"Volkswagen","color":"Black"},{"manufacturer":"BMW","color":"Grey"},{"manufacturer":"Audi","color":"White"}]}
-
Insert data
To insert data to the database , we’ll use the HTTP POST method .We should first define the route
$app->post('/newcar', 'addCar');
Then we should define our ‘addCar’ method. In this method we will start first by getting the parameters sent by the POST method
$manufacturer = $req->params('manufacturer');
$color = $req->params('color');
Then write our Sql query
$sql = "INSERT INTO car (`manufacturer`,`color`) VALUES (:manufacturer, :color);";
And finally , execute the query
$db = getDB();
$stmt = $db->prepare($sql);
$stmt->bindParam("manufacturer", $manufacturer);
$stmt->bindParam("color",$color);
$stmt->execute();
So , our ‘addCar’ method will be
function addCar() {
global $app;
$req = $app->request();
$manufacturer = $req->params('manufacturer');
$color = $req->params('color');
$sql = "INSERT INTO car (`manufacturer`,`color`) VALUES (:manufacturer, :color);";
try {
$db = getDB();
$stmt = $db->prepare($sql);
$stmt->bindParam("manufacturer", $manufacturer);
$stmt->bindParam("color",$color);
$stmt->execute();
$db = null;
} catch(PDOException $e) {
echo "Error !";
}
}
To test , run this command in your terminal (cmd.exe if you are using Windows)
curl --data "manufacturer=Mercedes&color=Black" http://localhost/WebService-SlimFramework/webservice.php/newcar
🎉 Congratulations ! the data was successfully added to the database
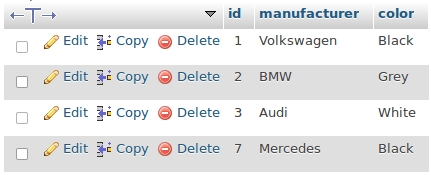
To conclude
Slim framework is a robust and secure micro framework that you can use for many projects that needs to connect to databases. It’s a framework that keeps getting better day after day , I hope that you liked it.
Thank you for following the tutorial , I hope you enjoyed it . Keep in touch to see my next tutorials
To download the full source code visit my Github repository here